React + TypeScript + Mobx 개발 환경 설정하기
2020 Aug 28
2 min read
2 min read
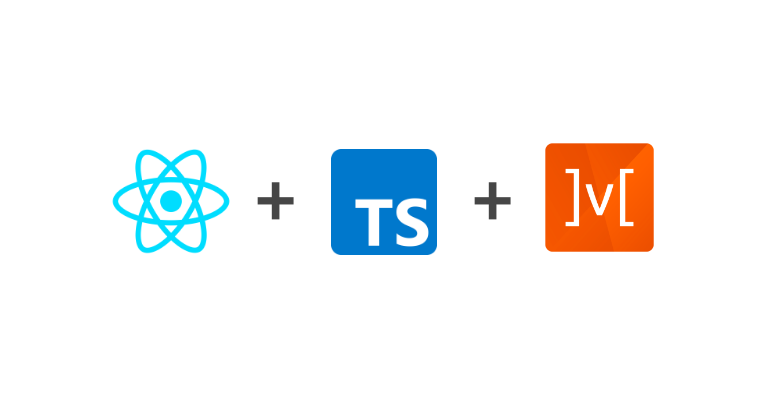
안녕하세요. 오늘은 React에 TypeScript와 mobx를 적용하여 개발 환경을 설정하는 방법을 알아보도록 하겠습니다.
SetUp
npx create-react-app <project-name> --typescript
우선 create-react-app으로 react + typescript 프로젝트를 만듭니다.
Mobx 설치
yarn add mobx mobx-react yarn add -D @babel/plugin-proposal-class-properties @babel/plugin-proposal-decorators core-decorators customize-cra react-app-rewired
또는
npm install mobx mobx-react npm install -D @babel/plugin-proposal-class-properties @babel/plugin-proposal-decorators core-decorators customize-cra react-app-rewired
mobx를 react에 적용하기 위해 패키지를 설치해 줍니다.
config-overrides.js 구성
root 디렉토리에 config-overrides.js
파일을 만들어 줍니다.
const { addDecoratorsLegacy, // decorator를 사용할 수 있도록 config 설정 disableEsLint, override } = require("customize-cra"); // 사용자 정의 webpack 설정 module.exports = { webpack: override(disableEsLint(), addDecoratorsLegacy()) };
config-overrides.js
에서 사용자 정의 webpack을 설정합니다.
package.json
package.json
의 script 부분을 다음과 같이 수정합니다.
"scripts": { "start": "react-app-rewired start", "build": "react-app-rewired build", "test": "react-app-rewired test", "eject": "react-scripts eject" },
tsconfig.json
tsconfig.json
에서 아래 내용을 추가합니다.
{ "compilerOptions": { "experimentalDecorators": true, } }
store 구성
CountStore.ts
src/stores
폴더를 만들고 CountStore.ts
를 만듭니다.
import { action, observable } from "mobx"; import { autobind } from "core-decorators"; @autobind class CountStore { @observable count: number = 0; @action handleCount = (change: number) => { this.count = change; }; } export default CountStore;
위와 같이 작성하면 count
는 전역 state가 되고, handleCount
는 매개변수로 받은 수를 count
에 저장합니다.
index.ts
src/stores
디렉토리에 index.ts
파일을 생성하고 다음과 같이 수정합니다.
import CountStore from "./CountStore"; const stores = { CountStore: new CountStore() }; export default stores;
index.tsx 수정
src/index.tsx
파일을 다음과 같이 수정합니다.
import React from "react"; import { Provider } from "mobx-react"; import ReactDOM from "react-dom"; import App from "./App"; import * as serviceWorker from "./serviceWorker"; import stores from "./stores"; ReactDOM.render( <Provider store={stores}> <React.StrictMode> <App /> </React.StrictMode> </Provider>, document.getElementById("root") ); // If you want your app to work offline and load faster, you can change // unregister() to register() below. Note this comes with some pitfalls. // Learn more about service workers: https://bit.ly/CRA-PWA serviceWorker.unregister();
마무리
위와 같이 설정 후에는 store를 사용할 컴포넌트에서 다음과 같이 작성하여 사용할 수 있습니다.
import { inject, observer } from "mobx-react"; import React from "react"; import CountStore from "./stores/CountStore"; interface CountProps { store?: StoreType; } interface StoreType { CountStore: CountStore; } const Count = inject("store")( observer(({ store }: CountProps) => { const { count, handleCount } = store!.CountStore; return <div>{count}</div>; }) ); export default Count;
오늘은 React에 TypeScript와 Mobx를 적용하는 방법을 알아보았습니다.
presentational component container component
의 구조를 지닌 React + TypeScript + Mobx 기본 설정이 되어있는 Github 링크를 남겨두도록 하겠습니다. 참고용으로 쓰시면 좋을 것 같습니다.